Damstra Learning Public API
API Endpoint
https://app.velpic.com/api/v2Version: 2.0
The Damstra Learning REST API provides programatic access to read and write Damstra Learning data. All responses are in JSON format
Getting Started ¶
Here’s a quick rundown on how to get started using the Damstra Learning API
Generate an API key
If you wish to use our public REST API to integrate with Damstra Learning, you will need an API Access Key to use in a header for authentication. To generate one, please follow these steps.
Please Note: Not all subscription levels have access to the integrations tab. If you do not see this tab, you can contact team@velpic.com to enquire about having integrations enabled
- First select the Integrations view on the left navigation of the Manage section.
- This will open up the integrations section in your main panel. The options you see may differ according to your subscription level.
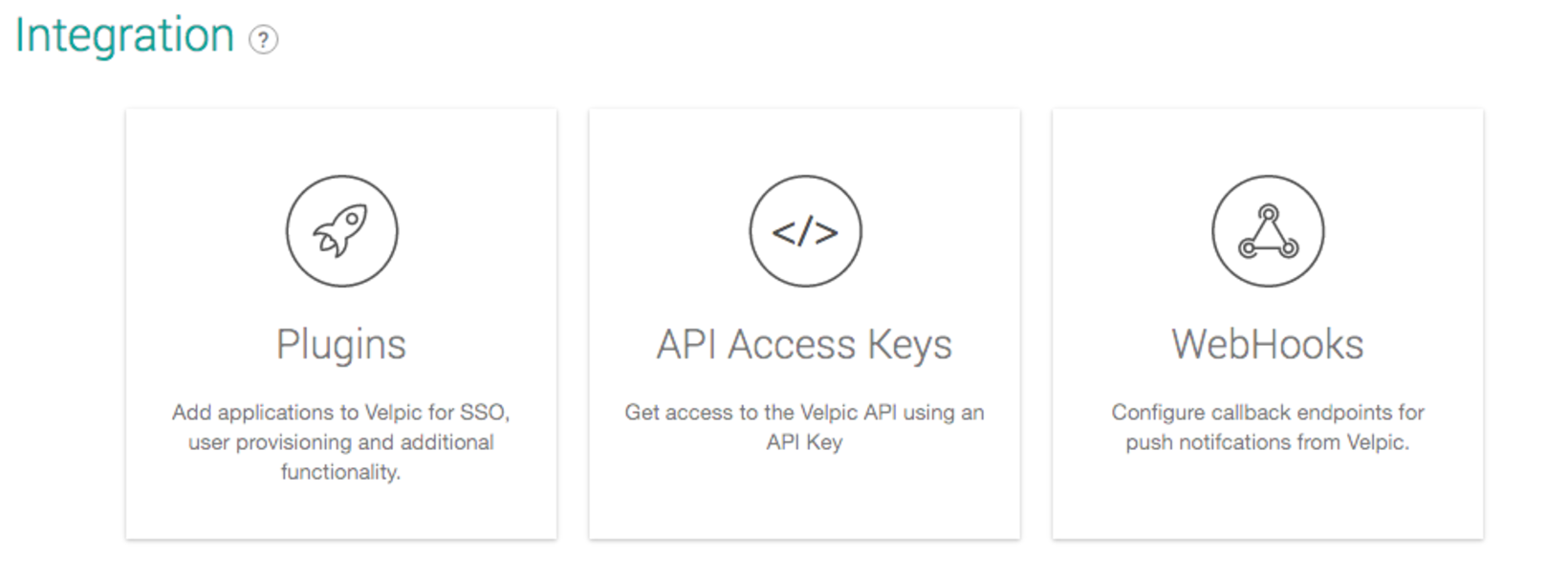
- Click API Access Keys that and you will see this view. On your first visit, you will not have an API Key yet.
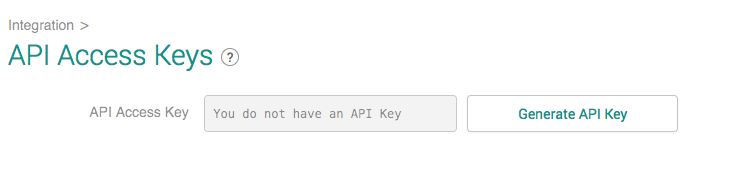
- Click on Generate API Key and the system will generate your first API Key. Keep this value secret, it is the value which authenticates your account when calling our REST api.
Regenerating your API key
If you wish to cycle it due to security concerns, etc, the click on the Regenerate API Key button. This will inactivate your current key and issue you a new one. Please keep in mind that this will break any integrations that you have written that are using the previous value. You can only have one active API Key at a time.
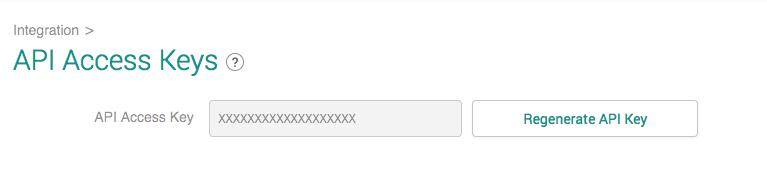
Using your API Key
Your access key is an alpha-numeric string that must be attached in the header of all requests as AccessKey. This key is secret and should be treated like a password.
"accesskey": "[ACCESS_KEY]"
Example Request – Get a list of trainees
Using cURL on Linux, Mac or Windows
Install cURL from https://curl.haxx.se/download.html then run the following command
curl -X GET -H "AccessKey: [ACCESS_KEY]" -H "Content-Type: application/json" "https://app.velpic.com/api/v2/user/?roleId=3"
This should return a set of JSON objects, similar to the following.
{
results:
[
{
userName: 'smithjohn',
firstName: 'John',
lastName: 'Smith',
emailAddress: 'jsmith@yourorganisation.com',
mobileNumber: '0412 345 678',
phoneNumber: '94123456',
lastLoggedInDate: '2016-09-05T06:45:53.000Z',
active: true,
employeeNumber: '',
clientStatusId: 56,
groups: [Object],
groupsSupervised: [],
roles: [Object],
clientId: 76,
timeZone: 'Australia/Perth',
id: 12345,
createdDate: '2015-11-19T05:04:43.000Z',
modifiedDate: '2016-09-05T06:45:53.000Z'
},
{
userName: 'katepostman',
firstName: 'Kate',
lastName: 'Postman',
emailAddress: 'kpostman@yourorganisation.com',
mobileNumber: '0498 765 432',
phoneNumber: '61524567',
lastLoggedInDate: '2016-09-05T04:42:49.000Z',
active: true,
employeeNumber: '',
clientStatusId: 56,
groups: [Object],
groupsSupervised: [],
roles: [Object],
clientId: 76,
timeZone: 'Australia/Perth',
id: 98765,
createdDate: '2015-11-19T08:03:51.000Z',
modifiedDate: '2016-09-05T04:42:49.000Z'
}
],
meta:
{
totalResultCount: 2,
startIndex: 0,
endIndex: 2,
createdDate: '2016-09-05T07:38:00.550Z',
modifiedDate: '2016-09-05T07:38:00.550Z'
},
createdDate: '2016-09-05T07:38:00.550Z',
modifiedDate: '2016-09-05T07:38:00.550Z'
}
Using Node.js
Install Node.js Download and install Node.js from https://nodejs.org/en/download/
Create a JavaScript file Create a file called GetTrainees.js and open it. In the file add the following code, replacing [ACCESS_KEY] with your access key:
var http = require("https");
var options = {
"method": "GET",
"hostname": "app.velpic.com",
"port": null,
"path": "/api/v2/user/?roleId=6&maxResults=100",
"headers": {
"accesskey": "[ACCESS_KEY]",
"content-type": "application/json"
}
};
var req = http.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function () {
var body = JSON.parse(Buffer.concat(chunks));
console.dir(body);
});
});
req.end();
This will return a set of users who are Trainees.
Running the request
In your terminal, navigate to the folder you saved GetTrainees.js in and then enter the command.
node GetTrainees.js
Webhooks ¶
WebHook integration provides Velpic with a way of communicating real-time information to third-party applications using HTTP callbacks.
Specification
WebHooks make HTTP POST requests to send data in real-time. This is advantageous over traditional API’s which require frequent polling, a process that can be less efficient. Messages are sent in JSON format.
Create a WebHook
WebHooks can be created through the Velpic App. To create a WebHook, follow the steps below
STEP 1: NAVIGATE TO THE INTEGRATION PAGE
In the app, navigate to the Integration tab under “Manage”
STEP 2: NAVIGATE TO THE WEBHOOKS PAGE
The options that you see here will differ according to your subscription level, but the one you want is “WebHooks”. Click on that
STEP 3: ADD A URI
Once in the integrations page, you will see an input for the WebHook URI. This is the address that the event posts will be sent to. Enter your desired URI here.
STEP 4: SELECT AN EVENT TYPE
You will also see a dropdown for the types of events for which messages will be posted. A list of these events and their payloads can be found in the Events section below.
After selecting your event type, click the add button. The WebHook setup is now complete.
You can now add more WebHooks to your account. There is no limit to the number of WebHooks you can set up.
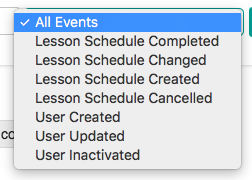
Delete a Webhook
WebHooks can be deleted from the WebHooks page. Simply click the trash icon beside the WebHook that you wish to delete.
When a WebHook is deleted, it is inactivated and will not generate ay new posts.
All Events
This event will trigger whenever any of the events in this section trigger.
Since there is no single event that will cause the WebHook to fire, there is no standard format for the post. Any handler would need to be able to accommodate all of the events below. However, all events have a name element which defines which event is fired
Lesson Schedule Completed ¶
Headers
Content-Type: application/json
Body
{
"name": "lesson.schedule.completed",
"lessonScheduleId": "1233",
"userId": "1023",
"timeStamp": "2017-01-16T01:33:27.012Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"lessonScheduleId": {
"type": "string"
},
"userId": {
"type": "string"
},
"timeStamp": {
"type": "string"
}
}
}
Lesson Schedule CompletedPOST/your-endpoint
This event fires when a user completes a lesson schedule
The fields in the body of the post are as follows;
key | description |
---|---|
name |
The name of the event. For this event it is always be “lesson.schedule.completed” |
lessonScheduleId |
The ID number of the lesson schedule that was completed |
userID |
The ID number of the user that completed the lesson schedule |
timeStamp |
The time at which the event was fired |
Lesson Schedule Changed ¶
Headers
Content-Type: application/json
Body
{
"name": "lesson.schedule.changed",
"scheduleDate": "2017-01-20T01:33:27.012Z",
"lessonScheduleId": "1233",
"userID": "1023",
"timeStamp": "2017-01-16T01:33:27.012Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"scheduleDate": {
"type": "string"
},
"lessonScheduleId": {
"type": "string"
},
"userID": {
"type": "string"
},
"timeStamp": {
"type": "string"
}
}
}
Lesson Schedule ChangedPOST/your-endpoint
This event fires when a lesson schedule is changed. If a schedule assigned to multiple users is changed, the event will fire for each user.
The fields in the body of the post are as follows;
key | description |
---|---|
name |
The name of the event. For this event it is always be “lesson.schedule.changed” |
scheduleDate |
The new start date for the schedule |
lessonScheduleId |
The ID number of the lesson schedule that was changed |
userID |
The ID number of the user that is assigned to the lesson schedule that was changed |
timeStamp |
The time at which the event was fired |
Lesson Schedule Created ¶
Headers
Content-Type: application/json
Body
{
"name": "lesson.schedule.created",
"lessonScheduleId": "1233",
"userID": "1023",
"timeStamp": "2017-01-16T01:33:27.012Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"lessonScheduleId": {
"type": "string"
},
"userID": {
"type": "string"
},
"timeStamp": {
"type": "string"
}
}
}
Lesson Schedule CreatedPOST/your-endpoint
This event fires when a lesson schedule is created. If a schedule assigned to multiple users is created, the event will fire for each user.
The fields in the body of the post are as follows;
key | description |
---|---|
name |
The name of the event. For this event it is always be “lesson.schedule.created” |
lessonScheduleId |
The ID number of the lesson schedule that was created |
userID |
The ID number of the user that is assigned to the new lesson schedule |
timeStamp |
The time at which the event was fired |
Lesson Schedule Cancelled ¶
Headers
Content-Type: application/json
Body
{
"name": "lesson.schedule.cancelled",
"lessonScheduleId": "1233",
"userID": "1023",
"cancellationReason": "SCHEDULE_CANCELLED_MANUALLY",
"timeStamp": "2017-01-16T01:33:27.012Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"lessonScheduleId": {
"type": "string"
},
"userID": {
"type": "string"
},
"cancellationReason": {
"type": "string"
},
"timeStamp": {
"type": "string"
}
}
}
Lesson Schedule CancelledPOST/your-endpoint
This event fires when a lesson schedule is cancelled. If a schedule assigned to multiple users is cancelled, the event will fire for each user.
The fields in the body of the post are as follows;
key | description |
---|---|
name |
The name of the event. For this event it is always be “lesson.schedule.cancelled” |
lessonScheduleId |
The ID number of the lesson schedule that was cancelled |
userID |
The ID number of the user that was assigned to the cancelled lesson schedule |
cancellationReason |
The reason the lesson was cancelled. See the table below for possible values |
timeStamp |
The time at which the event was fired |
User Account Created ¶
Headers
Content-Type: application/json
Body
{
"name": "user.account.created",
"firstName": "John",
"lastName": "Smith",
"userName": "j.smith",
"userId": "1023",
"timeStamp": "2017-01-16T01:33:27.012Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"firstName": {
"type": "string"
},
"lastName": {
"type": "string"
},
"userName": {
"type": "string"
},
"userId": {
"type": "string"
},
"timeStamp": {
"type": "string"
}
}
}
User Account CreatedPOST/your-endpoint
This event fires each time a user is created.
The fields in the body of the post are as follows;
key | description |
---|---|
name |
The name of the event. For this event it is always be “user.account.created” |
firstName |
The first name of the created user |
lastName |
The last name of the created user |
userName |
The userName of the created user |
userId |
The ID number of the created user |
timeStamp |
The time at which the event was fired |
User Settings Updated ¶
Headers
Content-Type: application/json
Body
{
"name": "user.settings.updated",
"userId": "1023",
"actingUserId": "1055",
"timeStamp": "2017-01-16T01:33:27.012Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"userId": {
"type": "string"
},
"actingUserId": {
"type": "string"
},
"timeStamp": {
"type": "string"
}
}
}
User Settings UpdatedPOST/your-endpoint
This event fires whenever a user’s settings are changed.
The fields in the body of the post are as follows;
key | description |
---|---|
name |
The name of the event. For this event it is always be “user.settings.updated” |
userId |
The ID number of the modified user |
actingUserId |
The ID number of user that changed the settings |
timeStamp |
The time at which the event was fired |
User Account Inactive ¶
Headers
Content-Type: application/json
Body
{
"name": "user.account.inactivate",
"userName": "j.smith",
"userId": "1023",
"timeStamp": "2017-01-16T01:33:27.012Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"userName": {
"type": "string"
},
"userId": {
"type": "string"
},
"timeStamp": {
"type": "string"
}
}
}
User Account InactivePOST/your-endpoint
This event fires whenever a user will be inactivated
The fields in the body of the post are as follows;
key | description |
---|---|
name |
The name of the event. For this event it is always be “user.account.inactivate” |
userName |
The username of the user that will be inactivated |
userId |
The ID number of user that will be inactivated |
timeStamp |
The time at which the event was fired |
User ¶
The user object contains all identifiying information about users within the platform.
Roles A user can have one or more of the following roles
Id | Role | Description |
---|---|---|
3 | ADMIN | Admins have access to the manage section and can create edit and update all objects in the system (users, lessons etc.) |
4 | EDITOR | Editors have the ability to create and edit lessons |
5 | SUPERVISOR | Supervisors are given additional abilities to update and manage the users in the group they are assigned to or any users they directly supervise |
6 | TRAINEE | A Trainee only has access to consume learning content and view results |
7 | OWNER | There is only ever one owner. |
8 | INSTRUCTOR | Intructors can run Instructor-led Training Sessions |
clientStatusId This is an id to indicate the users active status.
Id | Role | Description |
---|---|---|
56 | Active | a trainee who is currently in the organisation |
57 | Not Current | a trainee who is not currently active in the organisation. These people learning records are still available in Damstra Learning |
Search for Users ¶
Called to find users based on a number of criteria.
You can search for users by any of the paramaters. Specifying no paramaters will return users in a paged set.
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"id": 1,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"clientStatusId": 56,
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"roles": [
1
],
"timeZone": "Australia/Perth",
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"endIndex": 20
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "The list of users in a UserBasic object structure."
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"endIndex"
]
}
},
"required": [
"results"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
ListGET/user{?q,empId,emailAddress,startIndex,maxResults}
- q
string
(required) Example: abc(Optional) A string to query that matches to username, firstname or lastname of the user.
- empId
string
(required) Example: af09c(Optional) A exact match to the employee ID. If no match, then no user will be returned
- emailAddress
string
(required) Example: john@smith.com(Optional) A exact match to the email address for the user. If no match, then no user will be returned.
- startIndex
number
(required) Example: 0(Optional) The start index of the record set. Default is 0
- maxResults
number
(required) Example: 10(Optional) The maximum number of results to return for the search Default is 0
- activeValue
string
(required) Example: active(Optional) Filters list by user’s active status. One of
active
,inactive
orall
. Default is active
Get User ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"id": 1,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"clientStatusId": 56,
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"roles": [
1
],
"timeZone": "Australia/Perth",
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier"
},
"userName": {
"type": "string",
"description": "Users username"
},
"firstName": {
"type": "string",
"description": "Users first name"
},
"lastName": {
"type": "string",
"description": "Users last name"
},
"emailAddress": {
"type": "string",
"description": "The users email address"
},
"phoneNumber": {
"type": "string",
"description": "Phone number for the user"
},
"mobileNumber": {
"type": "string",
"description": "Mobile phone number for the user"
},
"employeeNumber": {
"type": "string",
"description": "Employee Number"
},
"clientStatusId": {
"type": "number",
"enum": [
56,
57
],
"description": "The active status Id for the user"
},
"groups": {
"type": "array",
"description": "The groups the user is a part of"
},
"groupsSupervised": {
"type": "array",
"description": "The groups a user supervises"
},
"roles": {
"type": "array",
"items": {
"type": "number",
"enum": [
3,
4,
5,
6,
7,
8
]
},
"description": "The roles for the user."
},
"timeZone": {
"type": "string",
"description": "the timezone for a user"
},
"createdDate": {
"type": "string",
"description": "The date the group was created"
},
"modifiedDate": {
"type": "string",
"description": "The date the group was created"
}
},
"required": [
"id",
"firstName",
"lastName",
"emailAddress",
"createdDate",
"modifiedDate"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
GetGET/user/{userId}
Called to retrieve a user based on ID
value | description |
---|---|
clientStatusId | An id that indicates the users active status. |
roles | Indicates the access level of the user. |
- userId
Number
(required) Example: 1The unique identifier for the user.
Create User ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"userName": "j.smith",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"sendWelcomeEmail": true,
"password": "xsr113sdgas53",
"roles": [
1
],
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"timeZone": "Australia/Perth"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"userName": {
"type": "string",
"description": "(Optional) a username to set for the new user. This will be automatically generated if none is supplied"
},
"firstName": {
"type": "string",
"description": "Firstname of the user"
},
"lastName": {
"type": "string",
"description": "Lastname of the user"
},
"emailAddress": {
"type": "string",
"description": "Email of the user"
},
"phoneNumber": {
"type": "string",
"description": "The phone number for the user"
},
"mobileNumber": {
"type": "string",
"description": "The mobile phone number for the user"
},
"employeeNumber": {
"type": "string",
"description": "The clients employee number for the user"
},
"sendWelcomeEmail": {
"type": "boolean",
"description": "(Optional) Set to configure user creation to send the welcome email. Is true by default."
},
"password": {
"type": "string",
"description": "(Optional) The plaintext password for the user"
},
"roles": {
"type": "array",
"items": {
"type": "number",
"enum": [
3,
4,
5,
6,
7,
8
]
},
"description": "(Optional) The roles to set for a user. By default users are created with the TRAINEE(6) role."
},
"groups": {
"type": "array",
"description": "The groups the user is a part of"
},
"groupsSupervised": {
"type": "array",
"description": "The groups a user supervises"
},
"timeZone": {
"type": "string",
"description": "(Optional) the timezone for a user. If not set, the timezone will default to the timezone set for the client"
}
}
}
Headers
Content-Type: application/json
Body
{
"id": 327027,
"createdDate": "2018-04-19T01:24:40.632Z",
"modifiedDate": "2018-04-19T01:24:40.637Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier for the user"
},
"createdDate": {
"type": "string",
"description": "The date the user was created"
},
"modifiedDate": {
"type": "string",
"description": "The date the user was last modified"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Update User ¶
Call to update a user within Damstra Learning for your organisation.
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"id": 1,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"password": "xsr113sdgas53",
"roles": [
1
],
"timeZone": "Australia/Perth"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier"
},
"userName": {
"type": "string",
"description": "Users username"
},
"firstName": {
"type": "string",
"description": "Users first name"
},
"lastName": {
"type": "string",
"description": "Users last name"
},
"emailAddress": {
"type": "string",
"description": "The users email address"
},
"phoneNumber": {
"type": "string",
"description": "Phone number for the user"
},
"mobileNumber": {
"type": "string",
"description": "Mobile phone number for the user"
},
"employeeNumber": {
"type": "string",
"description": "Employee Number (can be null when not used by company)"
},
"groups": {
"type": "array",
"description": "The groups the user is a part of"
},
"groupsSupervised": {
"type": "array",
"description": "The groups a user supervises"
},
"password": {
"type": "string",
"description": "The password for the user"
},
"roles": {
"type": "array",
"items": {
"type": "number",
"enum": [
3,
4,
5,
6,
7,
8
]
},
"description": "The roles for the user."
},
"timeZone": {
"type": "string",
"description": "the timezone for a user"
}
},
"required": [
"id",
"firstName",
"lastName",
"emailAddress",
"phoneNumber",
"mobileNumber",
"employeeNumber",
"roles",
"timeZone"
]
}
Headers
Content-Type: application/json
Body
{
"id": 1,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"clientStatusId": 56,
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"roles": [
1
],
"timeZone": "Australia/Perth",
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier"
},
"userName": {
"type": "string",
"description": "Users username"
},
"firstName": {
"type": "string",
"description": "Users first name"
},
"lastName": {
"type": "string",
"description": "Users last name"
},
"emailAddress": {
"type": "string",
"description": "The users email address"
},
"phoneNumber": {
"type": "string",
"description": "Phone number for the user"
},
"mobileNumber": {
"type": "string",
"description": "Mobile phone number for the user"
},
"employeeNumber": {
"type": "string",
"description": "Employee Number"
},
"clientStatusId": {
"type": "number",
"enum": [
56,
57
],
"description": "The active status Id for the user"
},
"groups": {
"type": "array",
"description": "The groups the user is a part of"
},
"groupsSupervised": {
"type": "array",
"description": "The groups a user supervises"
},
"roles": {
"type": "array",
"items": {
"type": "number",
"enum": [
3,
4,
5,
6,
7,
8
]
},
"description": "The roles for the user."
},
"timeZone": {
"type": "string",
"description": "the timezone for a user"
},
"createdDate": {
"type": "string",
"description": "The date the group was created"
},
"modifiedDate": {
"type": "string",
"description": "The date the group was created"
}
},
"required": [
"id",
"firstName",
"lastName",
"emailAddress",
"createdDate",
"modifiedDate"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Bulk Create User ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"users": [
{
"userName": "j.smith",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"roles": [
1
],
"timeZone": "Australia/Perth"
}
],
"mergeType": "none"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"users": {
"type": "array",
"description": "The list of users in a UserBasic object structure."
},
"mergeType": {
"type": "string",
"enum": [
"none",
"email",
"empNo"
],
"description": "Field to use as identifier to update an existing user"
}
},
"required": [
"users"
]
}
Headers
Content-Type: application/json
Body
[
{
"id": 1,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"clientStatusId": 56,
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"roles": [
1
],
"timeZone": "Australia/Perth",
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
]
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "array"
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Bulk CreatePOST/bulkUserImport
Called to bulk create or update users in Damstra Learning
value | description |
---|---|
roles | Indicates the access level of the user. If none is specified it will reset role to trainee. |
mergeType
can be used to identify if you’d like users to be merged based on a field.
Possible values for mergeType are;
value | description |
---|---|
empNo |
Will merge a user with another based on the employee number supplied |
email |
Will merge a user with another based on the email address supplied |
userName |
Will merge a user with another based on the user name supplied |
none |
No merge will occur. this is the default |
Activate User ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
ActivatePOST/user/{userId}/activate
Called to activate a user based on ID
- userId
Number
(required) Example: 1The unique identifier for the user.
Inactivate User ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
InactivatePOST/user/{userId}/inactivate
Called to inactivate a user based on ID
- userId
Number
(required) Example: 1The unique identifier for the user.
Generate Login URL ¶
Create a magic link url that provides automatic, username and password free account login when clicked.
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
touch_token_url
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Generate Login URLPOST/user/{userId}/generate_login_url{?expiresIn}
- userId
String
(required) Example: 1The unique identifier for the user.
- expiresIn
String
(required) Example: dayThe time the magic link expires (disabled, never, day, week, month, year)
Group ¶
APIs that allow for read and write of Groups.
A Damstra Learning group contains members and supervisors. Supervisors have the ability to perform manage functions on users in the group and view followup information. Automatic scheduling rules can be set for members of a group.
List Groups ¶
Headers
Content-Type: application/json
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"id": 1,
"name": "Dev Group",
"parentId": 1,
"description": "The Development Team",
"memberCount": 15,
"createdDate": "2015-10-29 15:28:59",
"clientId": 1
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"endIndex": 20
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "The result of groups."
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"endIndex"
]
}
},
"required": [
"results"
]
}
Headers
Content-Type: application/json
Body
{
"internalCode": 806,
"error": "Token not valid"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
ListGET/group{?q,startIndex,maxResults}
- q
string
(required) Example: myGroupThe query string for the group search. Will match to group name
- startIndex
number
(required) Example: 0The starting index to list from in the result set.
- maxResults
number
(required) Example: 20The maximum number of results to return.
Get ¶
Headers
Content-Type: application/json
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"id": 1,
"name": "Dev Group",
"parentId": 1,
"description": "The Development Team",
"memberCount": 15,
"createdDate": "2015-10-29 15:28:59",
"clientId": 1
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier"
},
"name": {
"type": "string",
"description": "The name of the group"
},
"parentId": {
"type": "number",
"description": "The id of the parent group"
},
"description": {
"type": "string",
"description": "The group description"
},
"memberCount": {
"type": "number",
"description": "The number of members in the group"
},
"createdDate": {
"type": "string",
"description": "The date the group was created"
},
"clientId": {
"type": "number",
"description": "The related client Id"
}
},
"required": [
"id",
"name",
"description",
"memberCount",
"createdDate",
"clientId"
]
}
Headers
Content-Type: application/json
Body
{
"internalCode": 806,
"error": "Token not valid"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"message":"No group found for that Id",
"reason":"No Group found",
"error":true,
"internalCode":TBD,
"statusCode":404
}
GetGET/group/{groupId}
Retrieve a group based on ID
- groupId
Number
(required) Example: 1234The group unique identifier.
Create Group ¶
Headers
Content-Type: application/json
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"name": "Dev Group",
"description": "The Development Team",
"parentId": 1
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string",
"description": "The name of the group"
},
"description": {
"type": "string",
"description": "The group description"
},
"parentId": {
"type": "number",
"description": "The id of the parent group"
}
},
"required": [
"name",
"description"
]
}
Headers
Content-Type: application/json
Body
{
"id": 1,
"name": "Dev Group",
"parentId": 1,
"description": "The Development Team",
"memberCount": 15,
"createdDate": "2015-10-29 15:28:59",
"clientId": 1
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier"
},
"name": {
"type": "string",
"description": "The name of the group"
},
"parentId": {
"type": "number",
"description": "The id of the parent group"
},
"description": {
"type": "string",
"description": "The group description"
},
"memberCount": {
"type": "number",
"description": "The number of members in the group"
},
"createdDate": {
"type": "string",
"description": "The date the group was created"
},
"clientId": {
"type": "number",
"description": "The related client Id"
}
},
"required": [
"id",
"name",
"description",
"memberCount",
"createdDate",
"clientId"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
CreatePOST/group
Called when a new group is created
Update ¶
Headers
Content-Type: application/json
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"name": "Dev Group",
"description": "The Development Team",
"parentId": 1
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string",
"description": "The name of the group"
},
"description": {
"type": "string",
"description": "The group description"
},
"parentId": {
"type": "number",
"description": "The id of the parent group"
}
},
"required": [
"name",
"description"
]
}
Headers
Content-Type: application/json
Body
{
"id": 1,
"name": "Dev Group",
"parentId": 1,
"description": "The Development Team",
"memberCount": 15,
"createdDate": "2015-10-29 15:28:59",
"clientId": 1
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier"
},
"name": {
"type": "string",
"description": "The name of the group"
},
"parentId": {
"type": "number",
"description": "The id of the parent group"
},
"description": {
"type": "string",
"description": "The group description"
},
"memberCount": {
"type": "number",
"description": "The number of members in the group"
},
"createdDate": {
"type": "string",
"description": "The date the group was created"
},
"clientId": {
"type": "number",
"description": "The related client Id"
}
},
"required": [
"id",
"name",
"description",
"memberCount",
"createdDate",
"clientId"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
UpdatePOST/group/{groupId}
When a groups information is updated
- groupId
Number
(required) Example: 1234The group unique identifier.
Get Users for a group ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"id": 1,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"clientStatusId": 56,
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"roles": [
1
],
"timeZone": "Australia/Perth",
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"endIndex": 20
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "a list of the members in the group"
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"endIndex"
],
"description": "Meta data information about the seach"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Get Group MembersGET/group/{groupId}/member{?startIndex,maxResults}
Called to get a list of users for a group
- groupId
Number
(required) Example: 1234The group unique identifier.
- startIndex
Number
(required) Example: 0The starting index to list from in the result set.
- maxResults
Number
(required) Example: 10The maximum number of results to return.
Add Members to Group ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"userIds": [
1,
2,
3,
4,
5,
6,
7,
8,
9,
10
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"userIds": {
"type": "array",
"description": "List of users ids to be added as group supervisors"
}
}
}
Headers
Content-Type: application/json
Body
HTTP/1.1 200 OK
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "Group with id 1 not found",
"reason": "Group not found",
"internalCode": 842,
"statusCode": 404
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "Description of the error"
},
"reason": {
"type": "string",
"description": "Reason for the error"
},
"internalCode": {
"type": "number",
"description": "internal velpic code for the error"
},
"statusCode": {
"type": "number",
"description": "The http code for the error"
}
}
}
Add MembersPOST/group/{groupId}/add_members
Called to set a user as a group member
- groupId
Number
(required) Example: 1234The group unique identifier.
Remove Members from Group ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"userIds": [
1,
2,
3,
4,
5,
6,
7,
8,
9,
10
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"userIds": {
"type": "array",
"description": "List of users ids to be removed"
}
}
}
Headers
Content-Type: application/json
Body
HTTP/1.1 200 OK
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "Group with id 1 not found",
"reason": "Group not found",
"internalCode": 842,
"statusCode": 404
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "Description of the error"
},
"reason": {
"type": "string",
"description": "Reason for the error"
},
"internalCode": {
"type": "number",
"description": "internal Damstra Learning code for the error"
},
"statusCode": {
"type": "number",
"description": "The http code for the error"
}
}
}
Remove MembersPOST/group/{groupId}/remove_members
Remove users (individual or multiple) from a group
- groupId
Number
(required) Example: 1234The group unique identifier.
Get Supervisors for a group ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"id": 1,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"emailAddress": "john.smith@gmail.com",
"phoneNumber": "0811112222",
"mobileNumber": "0411222333",
"employeeNumber": "E123",
"clientStatusId": 56,
"groups": [
{
"id": 1
}
],
"groupsSupervised": [
{
"id": 1
}
],
"roles": [
1
],
"timeZone": "Australia/Perth",
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"endIndex": 20
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "a list of the supervisors in the group"
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"endIndex"
],
"description": "Meta data information about the seach"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Get SupervisorsGET/group/{groupId}/supervisor{?startIndex,maxResults}
Called to get a list of the supervisor users for a group
- groupId
Number
(required) Example: 1234The group unique identifier.
- startIndex
Number
(required) Example: 0The starting index to list from in the result set.
- maxResults
Number
(required) Example: 10The maximum number of results to return.
Add Supervisor to Group ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"userIds": [
1,
2,
3,
4,
5,
6,
7,
8,
9,
10
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"userIds": {
"type": "array",
"description": "List of users ids to be added to the group"
}
}
}
Headers
Content-Type: application/json
Body
HTTP/1.1 200 OK
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "Group with id 1 not found",
"reason": "Group not found",
"internalCode": 842,
"statusCode": 404
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "Description of the error"
},
"reason": {
"type": "string",
"description": "Reason for the error"
},
"internalCode": {
"type": "number",
"description": "internal Damstra Learning code for the error"
},
"statusCode": {
"type": "number",
"description": "The http code for the error"
}
}
}
Add SupervisorsPOST/group/{groupId}/add_supervisors
Called to set a user as a group supervisor
- groupId
Number
(required) Example: 1234The group unique identifier.
Remove Supervisors from Group ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"userIds": [
1,
2,
3,
4,
5,
6,
7,
8,
9,
10
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"userIds": {
"type": "array",
"description": "List of supervisor users ids to be removed"
}
}
}
Headers
Content-Type: application/json
Body
HTTP/1.1 200 OK
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "Group with id 1 not found",
"reason": "Group not found",
"internalCode": 842,
"statusCode": 404
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "Description of the error"
},
"reason": {
"type": "string",
"description": "Reason for the error"
},
"internalCode": {
"type": "number",
"description": "internal velpic code for the error"
},
"statusCode": {
"type": "number",
"description": "The http code for the error"
}
}
}
Remove SupervisorsPOST/group/{groupId}/remove_supervisors
Remove supervisors (individual or multiple) from a group
- groupId
Number
(required) Example: 1234The group unique identifier.
Inactivate Group ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 500,
"error": "Internal Server Error"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
InactivatePOST/group/{groupId}/inactivate
Called to inactivate a group based on ID
- groupId
Number
(required) Example: 1The unique identifier for the group.
Lesson ¶
A Lesson is the learning content that can be scheduled for a trainee.
status
The lesson status can be one of the following
Value | Description |
---|---|
active |
A lesson that is published and can be scheduled |
inactive |
A lesson that was previously published, but inactivated. Cannot be scheduled. |
never |
A lesson that is being edited and never been published. Cannot be scheduled. |
rendering |
A lesson that is currently rendering. Cannot be scheduled. |
error |
A lesson that has errored during rendering. Cannot be scheduled. |
List Lessons ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"lessonVersionId": 1,
"lessonId": 1234,
"name": "Lesson One",
"duration": 133000,
"status": "active",
"inLibrary": false,
"version": 1,
"questionnaireType": 18,
"clientId": 1,
"lessonVersionCustomFields": [
{
"lessonVersionId": 22,
"customFieldId": 111,
"customFieldDescription": "Description of field",
"fieldId": "customField1",
"type": "string",
"value": "Field Value",
"id": 98765,
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
],
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000"
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"endIndex": 20
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "The list of lessons"
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"endIndex"
]
}
},
"required": [
"results"
]
}
Headers
Content-Type: application/json
Body
{
"internalCode": 806,
"error": "Token not valid"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
ListGET/lesson{?q,lessonIds,startIndex,maxResults,publishedStates}
- q
string
(required) Example: myGroupThe query string for the group search. Will match to group name
- lessonIds
array
(optional) Example: 1234,1235List of Lesson IDs to return
- startIndex
number
(required) Example: 0The starting index to list from in the result set.
- maxResults
number
(required) Example: 20The maximum number of results to return.
- publishedStates
string
(optional) Example: activeThe publishedStates value can be active,inactive,never,rendering,error.
List Lessons with Details ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"lessonVersionId": 1,
"lessonId": 1234,
"name": "Lesson One",
"published": 65,
"duration": 133000,
"status": "active",
"inLibrary": false,
"version": 1,
"clientId": 1
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"id": "null",
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000",
"endIndex": 20
},
"createdDate": "2015-10-29T15:28:59+0000",
"modifiedDate": "2015-10-29T15:28:59+0000",
"id": "null"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "The list of lessons"
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"id": {
"type": "string"
},
"createdDate": {
"type": "string",
"description": "The date the group was created"
},
"modifiedDate": {
"type": "string",
"description": "The date the group was created"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"createdDate",
"modifiedDate",
"endIndex"
]
},
"createdDate": {
"type": "string",
"description": "The date the group was created"
},
"modifiedDate": {
"type": "string",
"description": "The date the group was created"
},
"id": {
"type": "string"
}
},
"required": [
"results",
"createdDate",
"modifiedDate"
]
}
Headers
Content-Type: application/json
Body
{
"internalCode": 806,
"error": "Token not valid"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
List DetailsGET/lessondetails{?q,lessonIds,startIndex,maxResults,publishedStates}
- q
string
(required) Example: myGroupThe query string for the group search. Will match to group name
- lessonIds
array
(optional) Example: 1234,1235List of Lesson IDs to return
- startIndex
number
(required) Example: 0The starting index to list from in the result set.
- maxResults
number
(required) Example: 20The maximum number of results to return.
- publishedStates
string
(optional) Example: activeThe publishedStates value can be active,inactive,never,rendering,error.
Lesson Schedule ¶
Lesson Schedules capture a users current scheduled lesson and their status. A Lesson schedule can have one of the following status’;
Type | Description |
---|---|
scheduled | Lessons that are scheduled for the future but not available for completion yet. |
due | Lessons that are due to be completed |
overdue | Lessons that were not started past there scheduled date |
completed | Lessons that have been completed |
cancelled | Lessons that have been cancelled |
Get lesson schedule by Id ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"startDate": "2016-08-24T15:00:00.000Z",
"endDate": "2016-08-24T16:00:00.000Z",
"active": false,
"userId": 1234,
"clientId": 456788,
"clientName": "Your Company",
"lessonVersionId": 1216,
"lessonId": 123,
"version": 6,
"lessonName": "Lesson One",
"lessonDuration": 10000,
"lessonEvolution": 129,
"thumbnailId": 123456789,
"lessonThumbnail": "https://app.velpic.com/downloads/lessons/1216/thumbnail.png",
"lessonStartedDate": "2016-08-24T15:00:00.000Z",
"lessonCompletedDate": "2016-08-24T16:00:00.000Z",
"lessonCompletedBy": 12345,
"lessonCompletedByName": "John Smith",
"reminders": [],
"questionResults": [],
"questionCount": 8,
"questionnaireType": 19,
"userFirstName": "John",
"userLastName": "Smith",
"userUserName": "jsmith",
"userEmployeeNumber": "1356",
"userGroups": [],
"supervisors": [],
"published": 65,
"completionTypeId": 77,
"typeId": 81,
"questionnairePassRate": 80,
"progress": 10000,
"scheduleOverEmailAttempted": true,
"overdueEmailAttempted": false,
"movieId": 123987,
"moviePhysicalURI": "download/lessons/123/movieFile.mp4",
"movieSizeInBytes": 7849374,
"chapterCount": 5,
"id": 98765,
"createdDate": "2016-08-24T15:00:00.000Z",
"modifiedDate": "2016-08-24T15:00:00.000Z"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"startDate": {
"type": "string",
"description": "The scheduled start date of the lesson"
},
"endDate": {
"type": "string",
"description": "The due date of the lesson"
},
"active": {
"type": "boolean",
"description": "The active flag of the lesson"
},
"userId": {
"type": "number",
"description": "The UserId of the trainee assigned the lesson"
},
"clientId": {
"type": "number",
"description": "The ID of the client the trainee is part of"
},
"clientName": {
"type": "string",
"description": "The name of the client the trainee is part of"
},
"lessonVersionId": {
"type": "number",
"description": "the unique lesson version ID"
},
"lessonId": {
"type": "number",
"description": "The unique lesson ID"
},
"version": {
"type": "number",
"description": "The lesson version that the user has completed"
},
"lessonName": {
"type": "string",
"description": "The name of the lesson"
},
"lessonDuration": {
"type": "number",
"description": "The length of the lesson in milliseconds"
},
"lessonEvolution": {
"type": "number",
"description": "Indicates if the lesson is a module or video lesson"
},
"thumbnailId": {
"type": "number",
"description": "The thumbnail ID for the lesson"
},
"lessonThumbnail": {
"type": "string",
"description": "The URI for the lesson thumbnail"
},
"lessonStartedDate": {
"type": "string",
"description": "The date the trainee started the lesson"
},
"lessonCompletedDate": {
"type": "string",
"description": "The date the trainee finished the lesson"
},
"lessonCompletedBy": {
"type": "number",
"description": "The UserId of the user who completed the lesson"
},
"lessonCompletedByName": {
"type": "string",
"description": "The name of the user who completed the lesson"
},
"reminders": {
"description": "An array of the reminders sent to a trainee"
},
"questionResults": {
"description": "An array of the question results, includes questions and answers"
},
"questionCount": {
"type": "number",
"description": "The number of questions"
},
"questionnaireType": {
"type": "number",
"description": "The ID of the type of questionnaire"
},
"userFirstName": {
"type": "string",
"description": "The trainees first name"
},
"userLastName": {
"type": "string",
"description": "The trainees last name"
},
"userUserName": {
"type": "string",
"description": "The user name of the trainee"
},
"userEmployeeNumber": {
"type": "string",
"description": "The employee number of the trainee"
},
"userGroups": {
"description": "An array of the groups the trainee belongs to"
},
"supervisors": {
"description": "An array of the supervisors for this user or group"
},
"published": {
"type": "number",
"description": "The ID of the published status for a lesson"
},
"completionTypeId": {
"type": "number",
"description": "The ID of the method used to complete the lesson schedule"
},
"typeId": {
"type": "number",
"description": "The type ID of the lesson schedule method"
},
"questionnairePassRate": {
"type": "number",
"description": "The minimum pass rate of a test"
},
"progress": {
"type": "number",
"description": "The current position of the trainee through the lesson in milliseconds"
},
"scheduleOverEmailAttempted": {
"type": "boolean",
"description": "Shows if the trainee has received an email with the scheduled lesson linked"
},
"overdueEmailAttempted": {
"type": "boolean",
"description": "Shows if the trainee received an overdue email reminder"
},
"movieId": {
"type": "number",
"description": "The movie ID"
},
"moviePhysicalURI": {
"type": "string",
"description": "The movie URI location"
},
"movieSizeInBytes": {
"type": "number",
"description": "The movie file size in bytes"
},
"chapterCount": {
"type": "number",
"description": "The number of chapters in the lesson"
},
"id": {
"type": "number",
"description": "The id of the lesson schedule"
},
"createdDate": {
"type": "string",
"description": "The date the lesson schedule was created"
},
"modifiedDate": {
"type": "string",
"description": "The date the lesson schedule was last modified"
}
},
"required": [
"lessonId"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Get Lesson Schedule By IdGET/lesson_schedule/{lessonScheduleId}
Called to retrieve a lesson schedule by unique Id.
- lessonScheduleId
Number
(required) Example: 17The lesson schedule unique identifier.
Get User lesson schedules ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"lessonName": "Lesson One",
"lessonId": 123,
"lessonVersionId": 1216,
"version": 6,
"userId": 1234,
"startDate": "2016-08-24T15:00:00.000Z",
"endDate": "2016-08-24T16:00:00.000Z",
"lessonStartedDate": "2016-08-24T15:00:00.000Z",
"lessonCompletedDate": "2016-08-24T16:00:00.000Z",
"userFirstName": "John",
"userLastName": "Smith",
"lessonDuration": 10000,
"progress": 10000,
"chapterCount": 5,
"questionnaireType": 19,
"questionCount": 8,
"scheduleType": "automatic",
"typeId": 81,
"thumbnailId": 123456789,
"lessonThumbnail": "https://app.velpic.com/downloads/lessons/1216/thumbnail.png",
"status": "completed",
"statusId": 94,
"completionTypeId": 77,
"lessonCompletedBy": 12345,
"lessonCompletedByName": "John Smith",
"lessonEvolution": 129,
"id": 98765,
"createdDate": "2016-08-24T15:00:00.000Z",
"modifiedDate": "2016-08-24T15:00:00.000Z"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "The list of lessons"
}
},
"required": [
"results"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Get User Lesson SchedulesGET/user/{userId}/lesson_schedule{?status,startIndex,maxResults}
Called for a list of users lesson schedules.
- userId
Number
(required) Example: 4321The user unique identifier.
- startIndex
Number
(required) Example: 0The starting index to list from in the result set.
- maxResults
Number
(required) Example: 10The maximum number of results to return.
- status
string
(required) Example: completedThe status of the lesson schedules to return.
Choices:
all
scheduled
due
overdue
completed
cancelled
Schedule a User ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"lessonVersionIds": [
1,
4,
3
],
"scheduleDate": "2015-10-29T15:28:59+0000",
"gracePeriod": "14",
"gracePeriodUnit": "days"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"lessonVersionIds": {
"type": "array",
"description": "The name of the group"
},
"scheduleDate": {
"type": "string",
"description": "(Optional) the date to schedule a user to start a lesson. By default it is NOW(). the user will not be able to watch the lesson untl after the scheduleDate"
},
"gracePeriod": {
"type": "string",
"description": "(Optional) The time period in which the user has to complete the lesson. If defined, gracePeriodUnit is requred. Default is the default grace period for the account - configurable in global settings"
},
"gracePeriodUnit": {
"type": "string",
"description": "The grace period unit. Possible values are `days` and `months`"
}
},
"required": [
"lessonVersionIds",
"gracePeriod",
"gracePeriodUnit"
]
}
Headers
Content-Type: application/json
Body
{
"ids": [
1,
2,
3
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"ids": {
"type": "array",
"description": "An array of ids for any lesson schedules that were created."
}
}
}
Headers
Content-Type: application/json
Body
{
"error": "Lesson Schedule already exists"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Schedule a UserPOST/user/{userId}/lesson_schedule
Called to schedule a user for a lesson
Optional Paramaters
Paramater | Description |
---|---|
scheduleDate | The date to schedule a user to start a lesson. By default it is NOW(). the user will not be able to watch the lesson untl after the scheduleDate |
gracePeriod | The time period in which the user has to complete the lesson. If defined, gracePeriodUnit is requred. Default is the default grace period for the account - configurable in global settings |
gracePeriodUnit | The grace period unit. Possible values are days and months |
- userId
Number
(required) Example: 1234The user to schedule the lesson for
Manually Complete Lesson ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"lessonId": 100,
"lessonVersionId": 1216,
"completedDate": "2015-10-29T15:28:59+0000",
"manuallyCompletedByUserId": 1234
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"lessonId": {
"type": "number",
"description": "The lesson ID. Required if the lesson version ID is not supplied. Lesson must have a published lessonVersion."
},
"lessonVersionId": {
"type": "number",
"description": "The lesson version ID. Required if the lesson ID is not supplied. Must be published or previously been published."
},
"completedDate": {
"type": "string",
"description": "The date the lesson is completed"
},
"manuallyCompletedByUserId": {
"type": "number",
"description": "The user ID of the person who manually completed the lesson. We recommend creating a `Data Import` user or something similar for this. This user must be active."
}
},
"required": [
"completedDate",
"manuallyCompletedByUserId"
]
}
Headers
Content-Type: application/json
Body
{
"ids": [
869
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"ids": {
"type": "array",
"description": "The ID of the lesson schedule completed for the trainee"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Manually CompletePOST/lesson_schedule/{userId}/complete_lesson
Called to record a completed scheduled lesson for the specified user and lesson version
- userId
number
(required) Example: 1234The trainee’s user ID
Download a certificate for a passed completed lesson schedule ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/pdf
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 831,
"error": "You are not authorised to make this request"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Download a certificate for a passed completed lesson scheduleGET/lesson_schedule/{lessonScheduleId}/certificate
Called to schedule a user for a lesson
Optional Paramaters
- lessonScheduleId
Number
(required) Example: 4321The lesson schedule unique identifier.
ILT Session ¶
ILT Sessions contain information about the start and end time of a ILT Lesson session, the number of attendees, location and other attributes.
An ILT Session has one of the following states
Id | State | Description |
---|---|---|
1 | upcoming | The session hasn’t started |
2 | inprogress | The session is in progress |
3 | past | The session has been completed |
Status This is an id to indicate the ILT Session’s active status.
Id | Status | Description |
---|---|---|
1 | active | The session is currently able to be seen in the system |
2 | completed | The session has been completed |
3 | cancelled | The session was cancelled |
List ILT Sessions ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"id": 1,
"statusId": 1,
"instructorId": 731,
"instructorFirstName": "Test",
"instructorLastName": "Name",
"lessonId": 12,
"notes": "Test",
"numberOfPlaces": 1,
"startDatetime": "2018-05-19T01:00:06.000+0800",
"endDatetime": "2018-05-19T01:00:06.000+0800",
"location": "Here",
"createdDate": "2018-05-18T01:00:06.000+0800",
"modifiedDate": "2018-05-18T01:00:06.000+0800"
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"endIndex": 20
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "The result of ilt sessions."
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"endIndex"
]
}
},
"required": [
"results"
]
}
Headers
Content-Type: application/json
Body
{
"internalCode": 806,
"error": "Token not valid"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
ListGET/ilt_session{?startIndex,maxResults,lessonScheduleId,state,status,instructorId}
- startIndex
number
(required) Example: 0The starting index to list from in the result set.
- maxResults
number
(required) Example: 20The maximum number of results to return.
- lessonScheduleId
number
(optional) Example: 23The lesson schedule related to the ILT Session.
- state
number
(optional) Example: 2The id for the state of the ILT Session.
- status
number
(optional) Example: 2The id for the status of the ILT Session.
- instructorId
number
(optional) Example: 543The user id for the instructor of the ILT Session.
Get ILT Session ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"id": 1,
"instructorId": 731,
"statusId": 1,
"lessonId": 12,
"lessonName": "Test",
"instructorLed": true,
"notes": "Test",
"numberOfAttendees": 5,
"numberOfPlaces": 1,
"startDatetime": "2018-05-19T01:00:06.000+0800",
"endDatetime": "2018-05-19T01:00:06.000+0800",
"location": "Here",
"state": "past",
"attendanceRate": 90,
"passRate": 85,
"iltSessionCancellationTraineeNotificationEnabled": true,
"iltSessionCancellationInstructorNotificationEnabled": true,
"createdDate": "2018-05-18T01:00:06.000+0800",
"modifiedDate": "2018-05-18T01:00:06.000+0800"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier."
},
"instructorId": {
"type": "number",
"description": "The instructor id for the session."
},
"statusId": {
"type": "number",
"description": "The id for the status of the ILT Session."
},
"lessonId": {
"type": "number",
"description": "The lesson id that is related to this session."
},
"lessonName": {
"type": "string",
"description": "The name of the lesson related to this session."
},
"instructorLed": {
"type": "boolean",
"description": "The boolean for a lesson being an instructor led lesson."
},
"notes": {
"type": "string",
"description": "Text for any notes attached."
},
"numberOfAttendees": {
"type": "number",
"description": "The number of attendees for the session."
},
"numberOfPlaces": {
"type": "number",
"description": "The capacity of possible session attendees."
},
"startDatetime": {
"type": "string",
"description": "The start datetime of the session."
},
"endDatetime": {
"type": "string",
"description": "The finish datetime of the session."
},
"location": {
"type": "string",
"description": "The string for the location of the sesssion."
},
"state": {
"type": "string",
"description": "The string for the state of the session."
},
"attendanceRate": {
"type": "number",
"description": "The percentage of attendence for the session."
},
"passRate": {
"type": "number",
"description": "The percentage pass rate of attendees for the session."
},
"iltSessionCancellationTraineeNotificationEnabled": {
"type": "boolean",
"description": "The boolean switch for notifications on session cancellation sent to trainees."
},
"iltSessionCancellationInstructorNotificationEnabled": {
"type": "boolean",
"description": "The boolean switch for notifications on session cancellation sent to the instructor."
},
"createdDate": {
"type": "string",
"description": "The created datetime of the session."
},
"modifiedDate": {
"type": "string",
"description": "The last modified datetime of the session."
}
},
"required": [
"id",
"instructorId",
"startDatetime",
"endDatetime",
"location",
"state",
"createdDate",
"modifiedDate"
]
}
Headers
Content-Type: application/json
Body
{
"internalCode": 806,
"error": "Token not valid"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
GetGET/ilt_session/{iltSessionId}
Retrieve a ilt session based on ID
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
Create ILT Session ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"lessonId": 12,
"startDatetime": "2018-05-19T01:00:06.000+0800",
"endDatetime": "2018-05-19T01:00:06.000+0800",
"location": "Here",
"instructorId": 731,
"notes": "Test"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"lessonId": {
"type": "number",
"description": "The lesson id that is related to this session"
},
"startDatetime": {
"type": "string",
"description": "The start datetime of the session."
},
"endDatetime": {
"type": "string",
"description": "The finish datetime of the session."
},
"location": {
"type": "string",
"description": "The string for the location of the sesssion."
},
"instructorId": {
"type": "number",
"description": "The user id for the instructor of the session."
},
"notes": {
"type": "string",
"description": "Text for any notes attached."
}
},
"required": [
"startDatetime",
"endDatetime",
"location",
"instructorId"
]
}
Headers
Content-Type: application/json
Body
{
"id": 1,
"statusId": 1,
"instructorId": 731,
"instructorFirstName": "Test",
"instructorLastName": "Name",
"lessonId": 12,
"notes": "Test",
"numberOfPlaces": 1,
"startDatetime": "2018-05-19T01:00:06.000+0800",
"endDatetime": "2018-05-19T01:00:06.000+0800",
"location": "Here",
"createdDate": "2018-05-18T01:00:06.000+0800",
"modifiedDate": "2018-05-18T01:00:06.000+0800"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier."
},
"statusId": {
"type": "number",
"description": "The id for the status of the ILT Session."
},
"instructorId": {
"type": "number",
"description": "The user id for the instructor of the session."
},
"instructorFirstName": {
"type": "string",
"description": "The first name of the instructor for the session."
},
"instructorLastName": {
"type": "string",
"description": "The last name of the instructor for the session."
},
"lessonId": {
"type": "number",
"description": "The lesson id that is related to this session."
},
"notes": {
"type": "string",
"description": "Text for any notes attached."
},
"numberOfPlaces": {
"type": "number",
"description": "The capacity of possible session attendees."
},
"startDatetime": {
"type": "string",
"description": "The start datetime of the session."
},
"endDatetime": {
"type": "string",
"description": "The finish datetime of the session."
},
"location": {
"type": "string",
"description": "The string for the location of the sesssion."
},
"createdDate": {
"type": "string",
"description": "The created datetime of the session."
},
"modifiedDate": {
"type": "string",
"description": "The last modified datetime of the session."
}
},
"required": [
"id",
"instructorId",
"startDatetime",
"endDatetime",
"location",
"createdDate",
"modifiedDate"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
CreatePOST/ilt_session
Create a new ilt session
Update ILT Session ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"instructorId": 731,
"statusId": 1,
"notes": "Test",
"numberOfPlaces": 1,
"location": "Here"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"instructorId": {
"type": "number",
"description": "The user id for the instructor of the session."
},
"statusId": {
"type": "number",
"description": "The id for the status of the ILT Session."
},
"notes": {
"type": "string",
"description": "Text for any notes attached."
},
"numberOfPlaces": {
"type": "number",
"description": "The capacity of possible session attendees."
},
"location": {
"type": "string",
"description": "The string for the location of the sesssion."
}
},
"required": [
"instructorId",
"location"
]
}
Headers
Content-Type: application/json
Body
{
"id": 1,
"instructorId": 731,
"statusId": 1,
"lessonId": 12,
"lessonName": "Test",
"instructorLed": true,
"notes": "Test",
"numberOfAttendees": 5,
"numberOfPlaces": 1,
"startDatetime": "2018-05-19T01:00:06.000+0800",
"endDatetime": "2018-05-19T01:00:06.000+0800",
"location": "Here",
"state": "past",
"attendanceRate": 90,
"passRate": 85,
"iltSessionCancellationTraineeNotificationEnabled": true,
"iltSessionCancellationInstructorNotificationEnabled": true,
"createdDate": "2018-05-18T01:00:06.000+0800",
"modifiedDate": "2018-05-18T01:00:06.000+0800"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier."
},
"instructorId": {
"type": "number",
"description": "The instructor id for the session."
},
"statusId": {
"type": "number",
"description": "The id for the status of the ILT Session."
},
"lessonId": {
"type": "number",
"description": "The lesson id that is related to this session."
},
"lessonName": {
"type": "string",
"description": "The name of the lesson related to this session."
},
"instructorLed": {
"type": "boolean",
"description": "The boolean for a lesson being an instructor led lesson."
},
"notes": {
"type": "string",
"description": "Text for any notes attached."
},
"numberOfAttendees": {
"type": "number",
"description": "The number of attendees for the session."
},
"numberOfPlaces": {
"type": "number",
"description": "The capacity of possible session attendees."
},
"startDatetime": {
"type": "string",
"description": "The start datetime of the session."
},
"endDatetime": {
"type": "string",
"description": "The finish datetime of the session."
},
"location": {
"type": "string",
"description": "The string for the location of the sesssion."
},
"state": {
"type": "string",
"description": "The string for the state of the session."
},
"attendanceRate": {
"type": "number",
"description": "The percentage of attendence for the session."
},
"passRate": {
"type": "number",
"description": "The percentage pass rate of attendees for the session."
},
"iltSessionCancellationTraineeNotificationEnabled": {
"type": "boolean",
"description": "The boolean switch for notifications on session cancellation sent to trainees."
},
"iltSessionCancellationInstructorNotificationEnabled": {
"type": "boolean",
"description": "The boolean switch for notifications on session cancellation sent to the instructor."
},
"createdDate": {
"type": "string",
"description": "The created datetime of the session."
},
"modifiedDate": {
"type": "string",
"description": "The last modified datetime of the session."
}
},
"required": [
"id",
"instructorId",
"startDatetime",
"endDatetime",
"location",
"state",
"createdDate",
"modifiedDate"
]
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
UpdatePATCH/ilt_session/{iltSessionId}
Update an ilt session
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
Complete ILT Session ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Complete SessionPOST/ilt_session/{iltSessionId}/complete
Complete an ilt session. This endpoint will complete all lesson schedules related to it as well as update the session itself.
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
Cancel ILT Session ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"iltSessionCancellationTraineeNotificationEnabled": true,
"iltSessionCancellationInstructorNotificationEnabled": true
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"iltSessionCancellationTraineeNotificationEnabled": {
"type": "boolean",
"description": "The boolean switch for notifications on session cancellation sent to trainees."
},
"iltSessionCancellationInstructorNotificationEnabled": {
"type": "boolean",
"description": "The boolean switch for notifications on session cancellation sent to the instructor."
}
},
"required": [
"iltSessionCancellationTraineeNotificationEnabled",
"iltSessionCancellationInstructorNotificationEnabled"
]
}
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Cancel SessionPOST/ilt_session/{iltSessionId}/cancel_session
Cancel an ilt session
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
Bulk Register ILT Session ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"userIds": [
1
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"userIds": {
"type": "array",
"description": "The userIds to register to this session."
}
},
"required": [
"userIds"
]
}
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Bulk RegisterPOST/ilt_session/{iltSessionId}/bulk_register
Bulk register an ilt session.
The users will need to be scheduled to the lesson before registering
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
Bulk Deregister ILT Session ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Body
{
"userIds": [
1
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"userIds": {
"type": "array",
"description": "The userIds to register to this session."
}
},
"required": [
"userIds"
]
}
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Bulk DeregisterPOST/ilt_session/{iltSessionId}/bulk_deregister
Bulk deregister users for an ilt session.
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
List ILT Session Attendees ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"results": [
{
"id": 1,
"userId": 423,
"sessionId": 12,
"lessonScheduleId": 98765,
"userName": "Username",
"firstName": "John",
"lastName": "Smith",
"registeringUserId": 123,
"registeringUserFirstName": "Jane",
"registeringUserLastName": "Williams",
"passed": true,
"attended": true,
"createdDate": "2018-05-18T01:00:06.000+0800",
"modifiedDate": "2018-05-18T01:00:06.000+0800"
}
],
"meta": {
"totalResultCount": 20,
"startIndex": 0,
"endIndex": 20
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"results": {
"type": "array",
"description": "The list of attendees for an ilt session"
},
"meta": {
"type": "object",
"properties": {
"totalResultCount": {
"type": "number",
"description": "The total number of results in the search"
},
"startIndex": {
"type": "number",
"description": "The start index of the result set"
},
"endIndex": {
"type": "number",
"description": "The end index of the result set"
}
},
"required": [
"totalResultCount",
"startIndex",
"endIndex"
]
}
},
"required": [
"results"
]
}
Headers
Content-Type: application/json
Body
{
"internalCode": 806,
"error": "Token not valid"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
List AttendeesGET/ilt_session/{iltSessionId}/attendees
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
Save Attendees Progress ¶
Body
{
"attendees": [
{
"id": 1,
"passed": true,
"attended": true
}
]
}
Schema
{
"type": "object",
"properties": {
"attendees": {
"type": "array",
"items": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "Unique identifier."
},
"passed": {
"type": "boolean",
"description": "The boolean for an attendee passing the session."
},
"attended": {
"type": "boolean",
"description": "The boolean for an attendee attending the session."
}
},
"required": [
"id"
]
},
"description": "The list of attendees for an ilt session"
}
},
"required": [
"attendees"
],
"description": "Content-Type: application/json\n AccessKey: \"Your API Access Key\"",
"$schema": "http://json-schema.org/draft-04/schema#"
}
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Save Attendees ProgressPOST/ilt_session/{iltSessionId}/save_progress
Save attendees progress.
- iltSessionId
Number
(required) Example: 1234The session unique identifier.
Embed Player ¶
Get Embed url for Player ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"embedUrl": "https://app.velpic.com/#player/4321?embed=true&token=YThhZTYzMWYtOGY2ZS00ODFjLWI0MWMtNjM3N2UxMzhiNzkz"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"embedUrl": {
"type": "string",
"description": "The full url for use in the iframe embed code"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 840,
"error": "Lesson Schedule does not exist"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 837,
"error": "This lesson schedule is completed"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 836,
"error": "This lesson schedule is cancelled"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Get Embed url for PlayerGET/embed_player{?lessonScheduleId}
Returns a url for embeding the Velpic lesson player into a iframe
- lessonScheduleId
Number
(required) Example: 4321The lesson schedule unique identifier.
Get Embed url for Player ¶
Headers
Content-Type: application/json
AccessKey: "Your API Access Key"
Headers
Content-Type: application/json
Body
{
"embedUrl": "https://app.velpic.com/#player/4321?embed=true&token=YThhZTYzMWYtOGY2ZS00ODFjLWI0MWMtNjM3N2UxMzhiNzkz"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"embedUrl": {
"type": "string",
"description": "The full url for use in the iframe embed code"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "AccessKey does not exist",
"reason": "Invalid access key",
"internalCode": 806,
"error": "Invalid security token"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "A description of the error"
},
"reason": {
"type": "string",
"description": "The reason for the error"
},
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 840,
"error": "Lesson Schedule does not exist"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 837,
"error": "This lesson schedule is completed"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Headers
Content-Type: application/json
Body
{
"internalCode": 836,
"error": "This lesson schedule is cancelled"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"internalCode": {
"type": "number",
"description": "The internal status code of the error"
},
"error": {
"type": "string",
"description": "The error for the message"
}
}
}
Get Embed url for all lessonGET/embed_player/version/{lessonScheduleId}
Returns a url for embeding the Velpic lesson player into a iframe (includes all type of lesson)
- lessonScheduleId
Number
(required) Example: 4321The lesson schedule unique identifier.